State management
- ここの記述やコードは以下のサイト(およびその子サイト)の内容に基づいています
- flutterは宣言的で
UI=f(state)
という形で成り立っている - 状態が変わったら自動的にUIが再描画される
- ユーザが管理すべきなのは「任意の時点でUIを再構築するために必要なあらゆるデータ」
- このような状態はephemeral stateとapp stateに分けられる
- UI stateやlocal stateとも呼ばれる
- 1つのWidgetに納められる状態のこと
PageView
での現在のページや複雑なanimationの現在の進行状況、BottomNavigationBar
の現在選択されたtabなど
- このような場合は状態管理テクニックを使わず、
StatefiuWidget
を使えば良い
StatefulWidget
を使えば全ての状態を管理できるので- また判断によって
ephemeral state
もapp state
となることもある - というわけでケースバイケースなので、明確な区切りはない
- ここではproviderパッケージを使いシンプルなアプリの状態管理をする
- 以下のようなアプリ
- catalogとcartの2つのscreenを持つ
- catalogはcustom app barを持ち、scroll viewも持つ
- 以下がWidget Tree
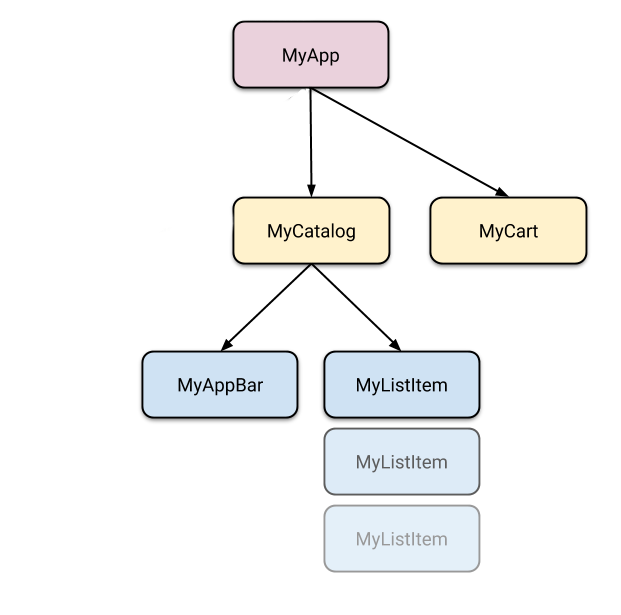
- flutterでは状態を使用するWidgetの上に配置する
- これはUIの変更は再構築となるので、
MyCart.updateWith(new)
ということはできない - つまりWidgetのメソッドを外部から呼び出して操作することはできない
// BAD: DO NOT DO THIS
// CartWidgetがitem一覧を持っていて、そこに更新したいitemを渡す感じ
void myTapHandler() {
var cartWidget = somehowGetMyCartWidget();
cartWidget.updateWith(item);
}
- 仮に動くようにできたとしても、以下のようなものにも対応しないといけない
// BAD: DO NOT DO THIS
Widget build(BuildContext context) {
return SomeWidget(
// The initial state of the cart.
);
}
void updateWith(Item item) {
// Somehow you need to change the UI from here.
}
- UIの現状を考慮しデータ更新していくのはバグを生みかねない
- flutterではcontentが変わったタイミングでWidgetが更新される
- というわけで以下のようにすべき
// GOOD
// まず親Widgetでcartの状態管理をする(cartModelを更新する)
void myTapHandler(BuildContext context) {
var cartModel = somehowGetMyCartModel(context);
cartModel.add(item);
}
// GOOD
// その上でCartWidgetないではそのモデルを参照する
Widget build(BuildContext context) {
var cartModel = somehowGetMyCartModel(context);
return SomeWidget(
// Just construct the UI once, using the current state of the cart.
// ···
);
}
- catalogのitemをユーザがタップしたらcartに加わりますが、cartは
MyListItem
の上にいるのにどうやってアクセスするのでしょうか? - 以下の感じでcallbackで上から指定することができる
@override
Widget build(BuildContext context) {
return SomeWidget(
// Construct the widget, passing it a reference to the method above.
MyListItem(myTapCallback),
);
}
void myTapCallback(Item item) {
print('user tapped on $item');
}
- 上記でも動くがいろいろな箇所で指定しないといけない
- flutterには
InheritedWidget
などで孫に渡したりできるが、より使いやすいprovider
を使うことにする
ChangeNotifier
はそのlistenerに変更を通知する- providerを使った
CartModel
は以下の感じ
class CartModel extends ChangeNotifier {
/// Internal, private state of the cart.
final List<Item> _items = [];
/// An unmodifiable view of the items in the cart.
UnmodifiableListView<Item> get items => UnmodifiableListView(_items);
/// The current total price of all items (assuming all items cost $42).
int get totalPrice => _items.length * 42;
/// Adds [item] to cart. This and [removeAll] are the only ways to modify the
/// cart from the outside.
void add(Item item) {
_items.add(item);
// This call tells the widgets that are listening to this model to rebuild.
notifyListeners();
}
/// Removes all items from the cart.
void removeAll() {
_items.clear();
// This call tells the widgets that are listening to this model to rebuild.
notifyListeners();
}
}
- 基本的にビジネスロジックに
notifyListeners
をつければいい - 以下のようにテストもできる
test('adding item increases total cost', () {
final cart = CartModel();
final startingPrice = cart.totalPrice;
var i = 0;
cart.addListener(() {
expect(cart.totalPrice, greaterThan(startingPrice));
i++;
});
cart.add(Item('Dash'));
expect(i, 1);
});
ChangeNotifier
をその子孫に提供するCartModel
の場合、MyCart
とMyCatalog
の両方の上のどこかに置く必要がある- というわけでMyAppを以下の感じにする
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => CartModel(),
child: const MyApp(),
),
);
}
- ここで
CartModel
をインスタンス化していて、lifecycleの管理はproviderがします - 複数個渡したいときは以下のように
MultiProvider
を使います
void main() {
runApp(
MultiProvider(
providers: [
ChangeNotifierProvider(create: (context) => CartModel()),
Provider(create: (context) => SomeOtherClass()),
],
child: const MyApp(),
),
);
}
return Consumer<CartModel>(
builder: (context, cart, child) {
return Text('Total price: ${cart.totalPrice}');
},
);
- まずアクセスしたいmodelの型指定が必要(今回は
CartModel
) Consumer
のbuilderはChangeNotifier
でnotifyListeners
が呼ばれたタイミングで呼ばれる- builderの引数は以下
- context…いつもの
- cart…
ChangeNotifier
のインスタンス - child…最適化のためにあるもので、Consumer下に重いけどモデルが変わっても変わらないWidgetがいる場合に使う
return Consumer<CartModel>(
builder: (context, cart, child) => Stack(
children: [
// Use SomeExpensiveWidget here, without rebuilding every time.
if (child != null) child,
Text('Total price: ${cart.totalPrice}'),
],
),
// Build the expensive widget here.
child: const SomeExpensiveWidget(),
);
- ベスプラとしては
Consumer
はできる限り奥におきたい
- cart全削除ボタンのように、アクセスはするけど変更通知が不要な場合は以下のようにできる
Provider.of<CartModel>(context, listen: false).removeAll();